Write a Program to draw Sine, Cosine and Tangent Curves
- #include<iostream.h>
- #include<conio.h>
- #include<graphics.h>
- #include<math.h>
- #include<stdio.h>
- #include<stdlib.h>
- #define pi 3.14
- class arc
- {
- float x, y, a, xm, ym;
- int i, ch;
- public:
- void get();
- void map();
- void sine();
- void cosine();
- void tangent();
- };
- void arc::get()
- {
- cout<<"\n ENTER THE MAXIMUM VALUE FOR Y";
- cin>>y;
- cout<<"\n MENU IS---------";
- cout<<"\n 1-> SINE CURVE";
- cout<<"\n 2->COSINE CURVE";
- cout<<"\n 3-> TANGENT CURVE";
- cout<<"\n 4-> EXIT";
- cout<<"\n ENTER YOUR CHOICE";
- cin>>ch;
- switch(ch)
- {
- case1:
- sine();
- break();
- case2:
- cosine();
- case3:
- tangent();
- case4:
- exit(0);
- }
- }
- void arc::sine()
- {
- cleardevice();
- xm=getmaxx()/2;
- ym=getmaxy()/2;
- line(xm, 0, xm, 2*ym);
- line(0, ym, 2 *xm, ym);
- outtextxy(0, ym, "X-AXIS");
- outtextxy(xm, 0, "Y-AXIS");
- for(x=-300;x<=300;x=x+0.5)
- {
- y=a*sin((pi*x)/180);
- putpixel(x+(320),-y+240,RED);
- }
- }
- void arc::cosine()
- {
- cleardevice();
- xm=getmaxx()/2;
- ym=getmaxy()/2;
- line(xm, 0, xm, 2*ym);
- line(0, ym, 2 *xm, ym);
- outtextxy(0, ym, "X-AXIS");
- outtextxy(xm, 0, "Y-AXIS");
- for(x=-300;x<=300;x=x+0.5)
- {
- y=a*cos((pi*x)/180);
- putpixel(x+(320),-y+240,RED);
- }
- }
- void arc :: map()
- {
- int gd=DETECT,gm;
- initgraph (&gd, &gm, "");
- int errorcode = graphresult();
- /*an error occurred */
- if (errorcode!=grOK)
- {
- printf("Graphics error: %s \n",grapherrormsg (errorcode));
- printf("Press and key to halt: ");
- getch();
- exit(1); /* terminate with an error code */
- }
- }
- void main()
- {
- class arc a;
- clrscr();
- a.map();
- a.get();
- getch();
- }
Output
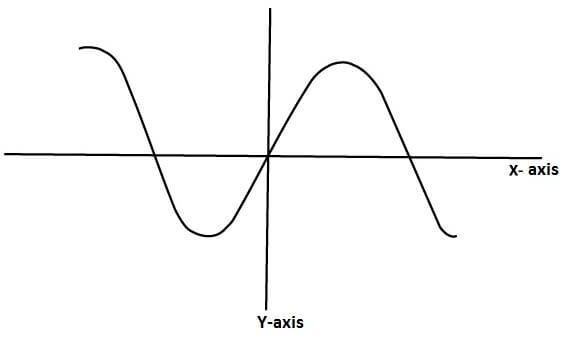